Networkx¶
Quick Guide for Networkx¶
Code by : Abolfazl Ziaeemehr
Creating Graphs¶
Basic Graph Types¶
NetworkX provides several types of graphs:
Graph: An undirected graph.
DiGraph: A directed graph.
MultiGraph: An undirected graph that can have multiple edges between nodes.
MultiDiGraph: A directed graph with multiple edges.
You can create an empty graph as follows:
[8]:
import numpy as np
import networkx as nx
G = nx.Graph() # or nx.DiGraph(), nx.MultiGraph(), nx.MultiDiGraph()
Adding Nodes and Edges¶
You can add nodes and edges to a graph using the following methods:
[13]:
# Add a single node
G.add_node(1)
# Add multiple nodes
G.add_nodes_from([2, 3])
# Add an edge between two nodes
G.add_edge(1, 2)
# Add multiple edges
G.add_edges_from([(2, 3), (3, 4)])
# get degree distribution
degrees = dict(G.degree())
degrees
[13]:
{1: 1, 2: 2, 3: 2, 4: 1}
Nodes can be any hashable Python object except None.
Node and Edge Attributes¶
You can also add attributes to nodes and edges:
[4]:
# Add node with attributes
G.add_node(4, color='red')
# Add edge with attributes
G.add_edge(1, 3, weight=4.2)
Graph Algorithms¶
NetworkX provides a wide range of graph algorithms, such as shortest path, clustering, and many others. For example, to find the shortest path using Dijkstra’s algorithm:
[5]:
# Create a weighted graph
G = nx.Graph()
edges = [('a', 'b', 0.3), ('b', 'c', 0.9), ('a', 'c', 0.5), ('c', 'd', 1.2)]
G.add_weighted_edges_from(edges)
# Find shortest path
path = nx.dijkstra_path(G, 'a', 'd')
print(path) # Output: ['a', 'c', 'd']
['a', 'c', 'd']
Visualization¶
NetworkX includes basic functionality for visualizing graphs, although it is primarily designed for graph analysis. You can use Matplotlib to draw graphs:
[6]:
import matplotlib.pyplot as plt
G = nx.complete_graph(5)
nx.draw(G, with_labels=True)
plt.show()
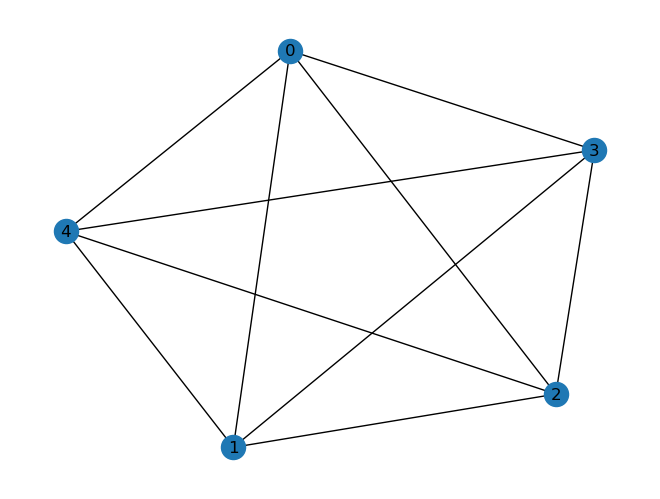
[ ]: